Fun with Zones of Control
I'm working on elaborating the movement rules by including an awareness of Zones of Control (ZOC). Every unit exerts a zone of control into the surrounding hexes. This has the effect of stopping movement, and triggers Defensive First Fire. I'm implementing movement in the prototype so that entering into movement mode identifies all hexes the unit can move to by highlighting those hexes. It's important to take ZOC's into account, especially since ZOC's stop movement and the rules do not permit movement from one ZOC to another.
This is what I had earlier today. There was a problem in the adjacent hex code...it did not always generate a list of adjacent hexes, resulting in the "jump" over the enemy units shown here.
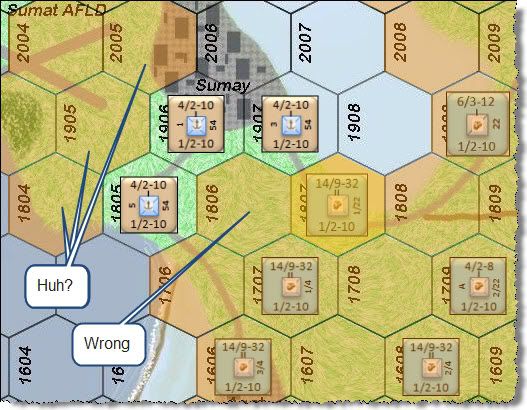
I fixed the adjacent hex code by providing the proper offsets and better controlling code:
This resulted in the following improvement in testing.
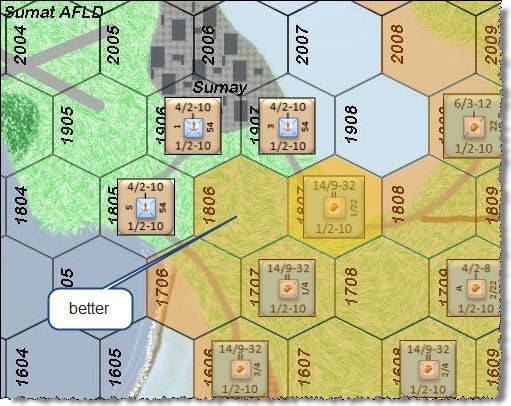
I did some more testing, setting up a situation in which hex 1806 should not be in the movement footprint due to the ZOC code. I still had some work to do:
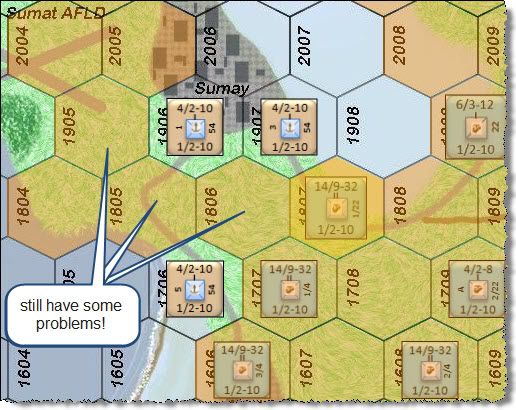
I had added the ZOC logic initially to the first set of adjacent hexes originating from the unit's starting location. I wondered whether I needed to also add that logic to the code that evaluated subsequent hexes further away from the starting point. This proved to be the case:
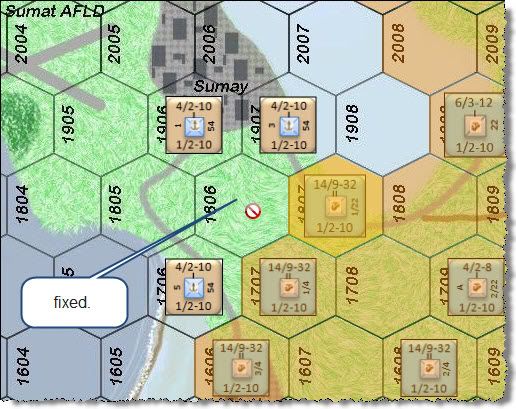
This finishes off the things on my list now for movement. I'm sure I've forgotten something, even cutting corners as I am for this prototype. Still, I think I have enough for now.
The next task I have is fire combat. This encompases Defensive First Fire, Offensive Fire, and Defensive Fire. This will take some doing.
I'm working on elaborating the movement rules by including an awareness of Zones of Control (ZOC). Every unit exerts a zone of control into the surrounding hexes. This has the effect of stopping movement, and triggers Defensive First Fire. I'm implementing movement in the prototype so that entering into movement mode identifies all hexes the unit can move to by highlighting those hexes. It's important to take ZOC's into account, especially since ZOC's stop movement and the rules do not permit movement from one ZOC to another.
This is what I had earlier today. There was a problem in the adjacent hex code...it did not always generate a list of adjacent hexes, resulting in the "jump" over the enemy units shown here.
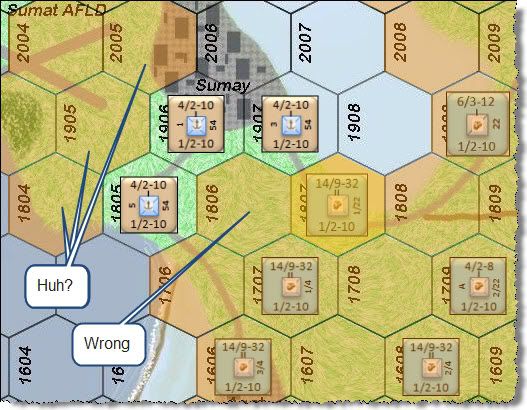
I fixed the adjacent hex code by providing the proper offsets and better controlling code:
public static List<Hex> AdjacentHexes(int x, int y)
{
List<Hex> adjacentHexes = new List<Hex>();
int[] XOffsets = { 1, 0, -1, -1, 0, 1 };
int[] evenYOffsets = { 1, 1, 1, 0, -1, 0 };
int[] oddYOffsets = { 0, 1, 0, -1, -1, -1 };
int[] YOffsets = (x % 2) == 0 ? evenYOffsets : oddYOffsets;
for (int offset = 0; offset < 6; offset++)
{
adjacentHexes.Add(Hexes.Where(h => h.X == x + XOffsets[offset] && h.Y == y + YOffsets[offset]).First());
}
List<Hex> adjacentHexesOnMap = new List<Hex>();
foreach (Hex h in adjacentHexes)
{
if (IsOnMap(h.Y, h.X))
{
adjacentHexesOnMap.Add(h);
}
}
return adjacentHexesOnMap;
}
This resulted in the following improvement in testing.
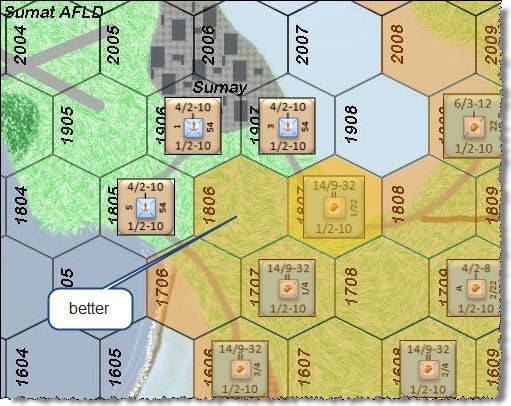
I did some more testing, setting up a situation in which hex 1806 should not be in the movement footprint due to the ZOC code. I still had some work to do:
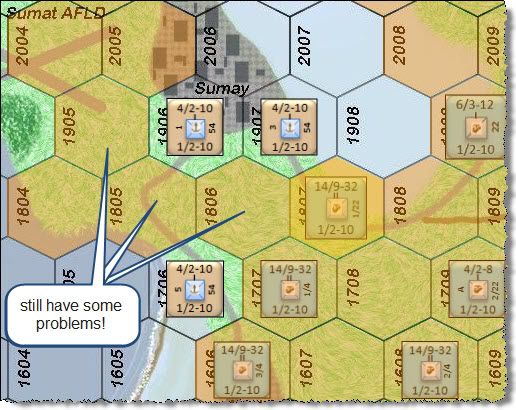
I had added the ZOC logic initially to the first set of adjacent hexes originating from the unit's starting location. I wondered whether I needed to also add that logic to the code that evaluated subsequent hexes further away from the starting point. This proved to be the case:
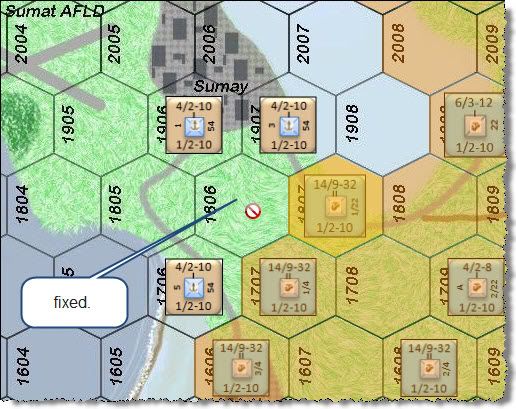
This finishes off the things on my list now for movement. I'm sure I've forgotten something, even cutting corners as I am for this prototype. Still, I think I have enough for now.
The next task I have is fire combat. This encompases Defensive First Fire, Offensive Fire, and Defensive Fire. This will take some doing.
0 Comments:
Post a Comment
<< Home